1. Ribbon?
Netflix가 만든 Software Load Balancer를 내장한 Library
2. Background
기존 L4, L7 장비를 통해 Server side Load balancing을 해주고 있다. H/W가 Load balancing을 해주기 때문에 WAS에서 처리해야 하는 SSL 등의 일을 대신 처리해주기도 하지만 다음과 같은 단점들이 있다.
- Switch 자체가 처리할 수 있는 요청에 한계가 있음
- Switch 증설 및 세팅 어려움, 고비용
- Switch 이중화 시, Active와 Stand-by 중 Stand-by는 사용되지 않으며 비싼 장비를 유휴장비로 방치
이러한 상황들을 극복하고자 나온것이 Client Side Load balancing이다.
그중 Spring Cloud 진형에서는 Netflix에서 개발한 Ribbon이 가장 유명하다.
3. 예제
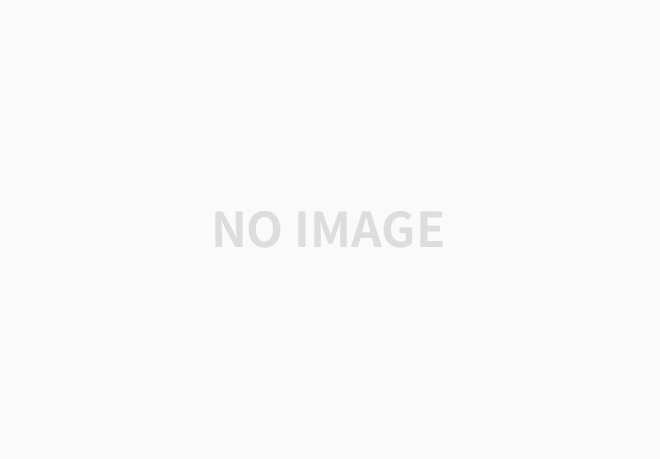
Ribbon Client에 세부 명세(ex. Ribbon Server list)를 하게되면, Server에 접근시 해당 명세를 보고 Client Load balancing을 진행한다.
3-1. Dependency
<!--pom.xml-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-ribbon</artifactId>
</dependency>
sping boot와 spring cloud에 대한 pom.xml작성 중 조심해야할 점은 parent에 cloud를 parent로 둘 수 없다는 것이며 이는 이전 글에 나와있음
3-2. Ribbon Client side
### application.yml
spring:
application:
name: webmain
server:
port: 8085
#api service name
api:
ribbon:
listOfServers: localhost:8090,localhost:9092,localhost:7077 #server 리스트
ServerListRefreshInterval: 15000 #15초 마다 서버 리스트 리프레시
/*
* Hystrix는 기본적으로 @Component / @Service를 찾고
* 그 안에 있는 @HystrixCommand를 찾아 동작한다.
*/
@Service("empInfoService")
@RibbonClient(name = "api", configuration = ApiConfiguration.class)
public class EmpInfoService {
private static final Logger logger = LoggerFactory.getLogger(EmpInfoService.class);
// 이 annotation은 spring cloud에게 아래 객체가 laod balancing 에 advantage를 받도록 말해준다.
@LoadBalanced
@Bean
public WebClient.Builder loadBalancedWebClientBuilder() {
return WebClient.builder();
}
@Autowired
private WebClient.Builder webClientBuilder;
/* annotaion을 통해 CircuitBreaker 기능 활성화
* 만약 Circuit이 Open이 될 경우 fallback method 수행
* default timeout : 1 sec
* HystrixProperty : https://github.com/Netflix/Hystrix/wiki/Configuration
*/
@HystrixCommand(commandKey = "info", fallbackMethod = "getEmpInfoFallback",
commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "3000"), // set timeout value 3 sec
@HystrixProperty(name = "circuitBreaker.errorThresholdPercentage", value = "10"), // error 비율이 10% 이상 발생하면 Circuit open
@HystrixProperty(name = "circuitBreaker.requestVolumeThreshold", value = "2") // 2회 이상 호출되면 통계 시작
})
public EmpInfo getEmpInfo() {
EmpInfo bodyEmpInfo = new EmpInfo();
bodyEmpInfo.setId(777);
bodyEmpInfo.setDomain("Jackpot");
WebClient webClient = webClientBuilder.baseUrl("http://api").build(); // yaml에 언급한 server list에서 load balancing
return webClient.post() // POST method
.uri("/api/getUserInfo") // baseUrl 이후 uri
.bodyValue(bodyEmpInfo) // set body value
.retrieve() // client message 전송
.bodyToMono(EmpInfo.class) // body type : EmpInfo
.block(); // await
}
// fallback method
private EmpInfo getEmpInfoFallback(Throwable t) {
logger.info(t.getMessage());
EmpInfo tmp = new EmpInfo();
tmp.setId(0);
tmp.setDomain("fallback123");
return tmp;
}
}
public class ApiConfiguration {
@Autowired
IClientConfig ribbonClientConfig;
// PingUrl은 언급된 url에 ping을 날려 server의 상태를 체크하는 것
// 여기에서는 아무것도 언급안했기 때문에 "/" path에 매핑됨
@Bean
public IPing ribbonPing(IClientConfig config){
return new PingUrl();
}
@Bean
public IRule ribbonRule(IClientConfig config) {
return new AvailabilityFilteringRule();
}
}
기본적으로 Ribbon은 서버에 연결하기 전에 Ping 체크를 하는 것으로 보여진다. 이를 위해 IPing이라는 bean을 사용하며, 따라서 Ribbon Server에는 Ping 체크를 위한 기본 path(http://API/)의 getMapping이 되어있어야 한다.
Reference
1. [Spring Cloud] 클라이언트 사이드 로드 밸런싱 (Client side Loadbalancing) 이란, 이경일, 2016. 9. 17 ,Kyoungil's Blog http://blog.leekyoungil.com/?p=259
Kyoungil's Blog
허접하지만 열심히 사는 잡부의 (공식적으로는 개발자인) 이야기...
blog.leekyoungil.com
2. Client Side Load Balancer: Ribbon, https://cloud.spring.io/spring-cloud-netflix/multi/multi_spring-cloud-ribbon.html
6. Client Side Load Balancer: Ribbon
Ribbon is a client-side load balancer that gives you a lot of control over the behavior of HTTP and TCP clients. Feign already uses Ribbon, so, if you use @FeignClient, this section also applies. A central concept in Ribbon is that of the named client. Eac
cloud.spring.io
3. Client Side Load Balancing with Ribbon and Spring Cloud, Spring IO, https://spring.io/guides/gs/client-side-load-balancing/
Spring
Level up your Java code and explore what Spring can do for you.
spring.io
'MSA > Spring' 카테고리의 다른 글
[Spring Cloud] Hystrix를 사용하여 Resilience한 시스템 구성하기 (0) | 2020.02.27 |
---|---|
[Spring Boot]WebClient를 이용한 POST 통신 (1) | 2020.02.26 |
[Spring Boot] 개발환경 세팅(visual studio code ver.) (0) | 2020.02.26 |